Desarrollo de software
Desbloqueo del desarrollo basado en pruebas (TDD): beneficios y estrategias que debe conocer
Anuncios
Desbloqueo del desarrollo basado en pruebas (TDD): beneficios y estrategias que debe conocer
If you’re a software developer, you’ve probably heard of Test-Driven Development (TDD). TDD is a software development technique that emphasizes writing tests before writing code. By doing so, developers gain a clear understanding of what the code should do before writing it, leading to better code quality and fewer bugs.
TDD can be a powerful technique for software development, but it requires a shift in mindset. Instead of writing code first and then writing tests to verify it, you write tests first and then write code to pass those tests. This approach can be challenging at first, but it can lead to significant benefits in the long term. In this article, we’ll explore the benefits of TDD and provide some strategies for unlocking its full potential.
Overview of Test-Driven Development
Test-Driven Development (TDD) is a software development approach that emphasizes writing tests before writing code. The concept revolves around the idea of creating small, manageable tests that define the desired behavior of the code. By doing so, developers gain a clear understanding of what the code should do before they write it.
The TDD process typically involves the following steps:
- Write a Test: First, you write a test that defines the expected behavior of a specific piece of code.
- Run the Test: The test is executed and, since the code doesn’t exist yet, it fails.
- Write the Code: You then write the code to pass the test.
- Run the Test Again: The test is executed again, and if it passes, the code is considered complete. If it fails, you refine the code until it passes.
The benefits of TDD are numerous. By writing tests before writing code, you can catch bugs early in the development process, which can save time and money in the long run. Additionally, TDD can help you write more modular, maintainable code that is easier to understand and modify.
Overall, TDD is a powerful technique that can help you develop high-quality software more efficiently. In the next section, we will explore the benefits of TDD in more detail.
The Fundamental Principles of TDD
Test-driven development (TDD) is a software development process that focuses on writing tests before writing the actual code. This approach has become increasingly popular in recent years, as it helps to ensure that the code is reliable, efficient, and well-structured. In this section, we will discuss the fundamental principles of TDD that make it such a powerful development methodology.
Red-Green-Refactor Cycle
The Red-Green-Refactor cycle is a fundamental principle of TDD. It involves three steps:
- Red: Write a failing test that defines the desired behavior of the code.
- Green: Write the simplest code that passes the test.
- Refactor: Improve the code without changing its behavior.
This cycle ensures that the code is tested thoroughly and that each test is written to test a specific behavior. By following this cycle, developers can be confident that their code is reliable and efficient.
Write Failing Tests First
In TDD, you write failing tests before writing the actual code. This approach ensures that the tests are written to test specific behaviors, and that the code is written to pass those tests. By writing failing tests first, you can be confident that your code is reliable and efficient.
Quick Feedback Loops
TDD emphasizes the importance of quick feedback loops. This means that developers should be able to get feedback on their code as quickly as possible. By writing tests first, developers can get feedback on their code almost immediately. This allows them to catch bugs and errors early in the development process, which can save time and money.
In conclusion, the fundamental principles of TDD are focused on ensuring that code is reliable, efficient, and well-structured. By following these principles, developers can be confident that their code is thoroughly tested and that it meets the desired requirements.
Benefits of TDD
Test-driven development (TDD) has numerous benefits that make it a popular software development approach. In this section, we will explore some of the key benefits of TDD.
Improved Code Quality
TDD encourages developers to write clean, modular, and well-tested code. By focusing on writing tests first, developers are forced to think about the design and architecture of their code upfront, leading to higher code quality. TDD also ensures that all code is tested, which reduces the likelihood of bugs and errors. By catching bugs early in the development process, developers can save time and resources that would have been spent fixing those bugs later.
Enhanced Documentation
TDD requires developers to write tests that define the desired behavior of the code. These tests serve as documentation and help developers to understand what the code should do. By writing tests first, developers can ensure that their code meets the requirements and specifications. This documentation is especially helpful when working on large projects or when multiple developers are working on the same codebase.
Better Design Decisions
TDD helps developers to develop the logic in their code. By starting tests with the simplest functionality first, developers can use them to guide their logic as they build up functionality. This helps to break a problem down into smaller, more manageable pieces, thus aiding the problem-solving process. TDD also encourages developers to write modular code that is easier to maintain and update over time.
Facilitation of Refactoring
Refactoring is the process of improving the design of existing code without changing its behavior. TDD makes refactoring easier by ensuring that all code is tested. Developers can make changes to the code with confidence, knowing that the tests will catch any errors or bugs. This makes it easier to improve the design of the code over time, leading to a more maintainable and scalable codebase.
In summary, TDD offers many benefits to developers and organizations. By improving code quality, enhancing documentation, aiding in better design decisions, and facilitating refactoring, TDD can help to create a more efficient and effective development process.
TDD Strategy and Implementation
When it comes to Test-Driven Development (TDD), choosing the right tools is crucial to the success of your project. You need to choose a testing framework that is easy to use, reliable, and efficient. Some popular testing frameworks for TDD include JUnit, NUnit, and TestNG. These frameworks provide a wide range of features that can help you write and run tests more effectively.
Choosing the Right Tools
JUnit is a popular testing framework for Java-based projects. It provides a simple and easy-to-use interface for writing and running tests. NUnit is a similar framework for .NET-based projects. It has a wide range of features that can help you write and run tests more efficiently. TestNG is another popular testing framework for Java-based projects. It provides advanced features such as parallel testing, data-driven testing, and test configuration.
Test Isolation
One of the key benefits of TDD is the ability to isolate tests. By isolating tests, you can ensure that each test is independent of the others. This means that if one test fails, it will not affect the other tests. To achieve test isolation, you need to use techniques such as dependency injection and mocking.
Mocking and Stubbing Techniques
Mocking and stubbing are two techniques that can help you achieve test isolation. Mocking involves creating a fake object that mimics the behavior of a real object. This allows you to test code that depends on the real object without actually using the real object. Stubbing involves creating a simplified version of a real object that provides only the functionality that is needed for the test. This allows you to test code that depends on the real object without using the entire object.
In summary, implementing TDD in your project requires careful consideration of the tools you use, and the techniques you employ to achieve test isolation. By choosing the right tools and techniques, you can ensure that your tests are reliable, efficient, and effective.
Common Challenges in TDD
Test-Driven Development (TDD) has many benefits, but it also presents some challenges that developers must overcome to be successful. In this section, we will discuss some of the most common challenges and strategies for dealing with them.
Dealing with Legacy Code
One of the biggest challenges in TDD is dealing with legacy code. Legacy code is code that was written before TDD was adopted, and it may not be easy to test. Legacy code may have complex dependencies, and it may be difficult to isolate the code that needs to be tested.
To deal with legacy code, you can start by identifying the most critical parts of the codebase and writing tests for those parts. You can also use tools like dependency injection to isolate the code that needs to be tested. Additionally, you can refactor the code to make it more testable.
Handling Complex Dependencies
Another challenge in TDD is handling complex dependencies. Complex dependencies are dependencies that are difficult to mock or stub. For example, a database connection or a network connection may be difficult to mock.
To handle complex dependencies, you can use tools like mocking frameworks to create mock objects that simulate the behavior of the real objects. You can also use dependency injection to replace the real objects with mock objects during testing.
Test Maintenance Overhead
Finally, TDD can sometimes lead to test maintenance overhead. As the codebase grows, the number of tests can become unmanageable. Additionally, changes to the codebase may require changes to the tests.
To minimize test maintenance overhead, you can write tests that are as simple as possible. You can also use tools like code coverage analysis to identify tests that are not necessary or redundant. Additionally, you can refactor the codebase to make it more modular and easier to test.
Effective Test Writing for TDD
When it comes to Test-Driven Development (TDD), writing effective tests is crucial. Effective tests should be granular, focus on behavior rather than implementation, and avoid being fragile. In this section, we’ll explore these concepts in detail.
Test Granularity
Tests should be granular, meaning that they should test a single behavior or function. This approach allows you to isolate and fix bugs more easily. It also makes it easier to maintain and refactor your codebase. When writing tests, you should aim to test the smallest unit of functionality possible.
Behavior vs. Implementation Testing
When writing tests, it’s important to focus on testing behavior rather than implementation. Behavior testing focuses on what the code should do, while implementation testing focuses on how the code does it. Behavior testing is more resilient to changes in implementation, making it more valuable in the long run. By focusing on behavior, you can ensure that your code is doing what it’s supposed to do, regardless of how it’s implemented.
Avoiding Fragile Tests
Fragile tests are tests that break easily due to changes in the codebase. To avoid fragile tests, you should aim to write tests that are independent of each other. This means that changing one test should not affect the outcome of another test. You should also avoid testing implementation details, as this can lead to fragile tests. Instead, focus on testing behavior, as mentioned earlier.
In summary, effective test writing for TDD requires tests that are granular, focus on behavior rather than implementation, and avoid being fragile. By following these principles, you can ensure that your tests are valuable, maintainable, and resilient to changes in your codebase.
TDD in Different Programming Paradigms
Test-Driven Development (TDD) can be applied to different programming paradigms. Here are some examples of how TDD can be used in different paradigms:
Object-Oriented TDD
Object-Oriented Programming (OOP) is a popular programming paradigm that emphasizes the use of objects and classes. TDD can be used in OOP to ensure that each class and object behaves as expected. By writing tests before writing the code, you can ensure that each object and class is tested thoroughly and that any bugs are caught early in the development process.
Functional TDD
Functional Programming (FP) is a programming paradigm that emphasizes the use of functions. TDD can be used in FP to ensure that each function behaves as expected. By writing tests before writing the code, you can ensure that each function is tested thoroughly and that any bugs are caught early in the development process.
Procedural TDD
Procedural Programming is a programming paradigm that emphasizes the use of procedures or subroutines. TDD can be used in procedural programming to ensure that each procedure or subroutine behaves as expected. By writing tests before writing the code, you can ensure that each procedure or subroutine is tested thoroughly and that any bugs are caught early in the development process.
Overall, TDD can be used in any programming paradigm to ensure that each piece of code behaves as expected and that any bugs are caught early in the development process.
Integrating TDD with Continuous Integration
When it comes to software development, integrating TDD with Continuous Integration (CI) can have significant benefits. By automating the testing process, you can save time and reduce the risk of errors. Here are some strategies for integrating TDD with CI:
Automated Test Suites
Automated test suites can be used to run tests automatically whenever code is changed. This can help ensure that new code does not break existing functionality. By using automated test suites, you can also catch errors early in the development process, which can save time and reduce costs.
Continuous Feedback
Continuous feedback is an essential part of the TDD and CI process. By providing developers with feedback on their code, they can quickly identify any errors and make the necessary changes. This can help ensure that the code is of high quality and meets the requirements of the project.
In conclusion, integrating TDD with CI can have significant benefits for software development. By automating the testing process and providing continuous feedback, you can save time, reduce costs, and ensure that the code is of high quality.
Scaling TDD for Large Projects
When it comes to scaling TDD for large projects, there are several strategies that you can use to ensure that your team is working efficiently and effectively. In this section, we’ll explore some of the most effective strategies for scaling TDD.
Modular Design
One of the most important strategies for scaling TDD is to use a modular design approach. This means breaking your code up into smaller, more manageable modules that can be tested independently. By doing this, you can ensure that your team is able to work on different parts of the project simultaneously, without interfering with each other’s work.
Team Coordination
Another important aspect of scaling TDD is team coordination. This means ensuring that your team is working together effectively, communicating regularly, and sharing knowledge and resources. By doing this, you can ensure that everyone is on the same page and that your project is moving forward smoothly.
Advanced Tooling
Finally, using advanced tooling can also help you scale TDD for large projects. This includes tools like automated testing frameworks, continuous integration tools, and more. By using these tools, you can automate many of the repetitive tasks involved in TDD, freeing up your team to focus on more important tasks.
Overall, scaling TDD for large projects requires a combination of careful planning, effective team coordination, and the right tools and strategies. By following these tips, you can ensure that your team is able to work efficiently and effectively, even on the largest and most complex projects.
Case Studies: TDD Success Stories
TDD has been adopted by many organizations and has helped them to improve their software development process. Here are a few examples of companies that have successfully implemented TDD:
Google is one of the most well-known companies that have implemented TDD. The company has a dedicated team of developers who are responsible for writing automated tests. By following the TDD approach, Google has been able to ensure that its code is well-tested and robust. One example of successful TDD implementation at Google is the development of the Google Testing Blog.
Microsoft
Microsoft has also adopted TDD in its software development process. The company has a team of developers who are responsible for writing automated tests. By following the TDD approach, Microsoft has been able to ensure that its code is well-tested and that any bugs are caught early in the development process. This has helped the company to release high-quality software products.
IBM
IBM has also implemented TDD in its software development process. The company has a team of developers who are responsible for writing automated tests. By following the TDD approach, IBM has been able to ensure that its code is well-tested and that any bugs are caught early in the development process. This has helped the company to reduce the time and cost of development.
Conclusión
These are just a few examples of companies that have successfully implemented TDD in their software development process. By adopting TDD, these companies have been able to improve the quality of their code, reduce the time and cost of development, and ensure that their software products are well-tested and robust.
Future of TDD
As the software development industry continues to evolve, Test-Driven Development (TDD) is expected to play a significant role in shaping the future of software engineering. Here are some emerging trends and how TDD is likely to impact next-gen technologies.
Emerging Trends
1. Agile Development
Agile development is an iterative approach to software development that emphasizes collaboration, flexibility, and customer satisfaction. TDD is a natural fit for agile development because it allows developers to write code that meets customer requirements and is easy to maintain.
2. DevOps
DevOps is a set of practices that combines software development and IT operations to shorten the systems development life cycle while delivering features, fixes, and updates frequently. TDD can help DevOps teams to automate testing and ensure that code changes are safe and reliable.
3. Cloud Computing
Cloud computing is a model that enables on-demand access to a shared pool of computing resources, including servers, storage, and applications. TDD can help cloud computing providers to ensure that their services are reliable, scalable, and secure.
TDD and Next-Gen Technologies
1. Machine Learning
Machine learning is a subset of artificial intelligence that allows computers to learn from data without being explicitly programmed. TDD can help machine learning developers to test their models and ensure that they are accurate and reliable.
2. Blockchain
Blockchain is a distributed ledger technology that enables secure and transparent transactions between parties without the need for intermediaries. TDD can help blockchain developers to test their smart contracts and ensure that they are secure and free from vulnerabilities.
3. Quantum Computing
Quantum computing is a new paradigm in computing that uses quantum-mechanical phenomena, such as superposition and entanglement, to perform operations on data. TDD can help quantum computing developers to test their algorithms and ensure that they are correct and efficient.
In conclusion, TDD is a powerful software development approach that is likely to shape the future of software engineering. By embracing TDD and keeping up with emerging trends and next-gen technologies, you can stay ahead of the curve and deliver high-quality software that meets customer requirements and exceeds expectations.
Preguntas frecuentes
How does Test-Driven Development (TDD) enhance code quality?
TDD requires developers to write tests before writing code. This approach helps to ensure that code meets the desired requirements and specifications. By writing tests first, developers are forced to think about the design and architecture of their code upfront, leading to higher code quality. Additionally, TDD encourages developers to write cleaner, more modular, and well-tested code. The result is software that is more reliable, maintainable, and easier to modify.
What are the key differences between TDD and traditional testing methods?
The key difference between TDD and traditional testing methods is the order in which tests are written. In traditional testing methods, tests are written after the code has been written. In contrast, TDD requires tests to be written before the code is written. This approach helps to ensure that code meets the desired requirements and specifications. Additionally, TDD encourages developers to write cleaner, more modular, and well-tested code.
In what ways can TDD facilitate better design in software development?
TDD encourages developers to write code that is modular, testable, and reusable. By writing tests first, developers are forced to think about the design and architecture of their code upfront. This approach leads to better software design, as developers are more likely to create code that is modular, testable, and reusable. Additionally, TDD helps to ensure that code meets the desired requirements and specifications.
How does TDD contribute to Agile methodologies?
TDD is a key part of Agile methodologies. It helps to ensure that code meets the desired requirements and specifications. Additionally, TDD encourages developers to write cleaner, more modular, and well-tested code. This approach leads to better software design, as developers are more likely to create code that is modular, testable, and reusable. TDD also helps to facilitate continuous integration and continuous delivery, which are key components of Agile methodologies.
What are the challenges and common pitfalls when implementing TDD?
One of the biggest challenges when implementing TDD is getting developers to buy into the process. TDD requires a shift in mindset and approach, which can be difficult for some developers to embrace. Additionally, TDD can be time-consuming, especially at the beginning of a project. Another common pitfall is writing tests that are too complex or too tightly coupled to the code. This can lead to brittle tests that are difficult to maintain.
Can TDD be integrated with Behavior-Driven Development (BDD), and if so, how?
Yes, TDD can be integrated with Behavior-Driven Development (BDD). BDD is an extension of TDD that focuses on the behavior of the system rather than the implementation details. BDD uses a natural language syntax to describe the behavior of the system, which can make it easier for non-technical stakeholders to understand. TDD and BDD can be used together to create a comprehensive testing strategy that covers both the behavior and implementation of the system.
Tendencias
Dilemas tecnológicos éticos: cómo afrontar los desafíos culturales y sociales en la era digital
Continúe Leyendo
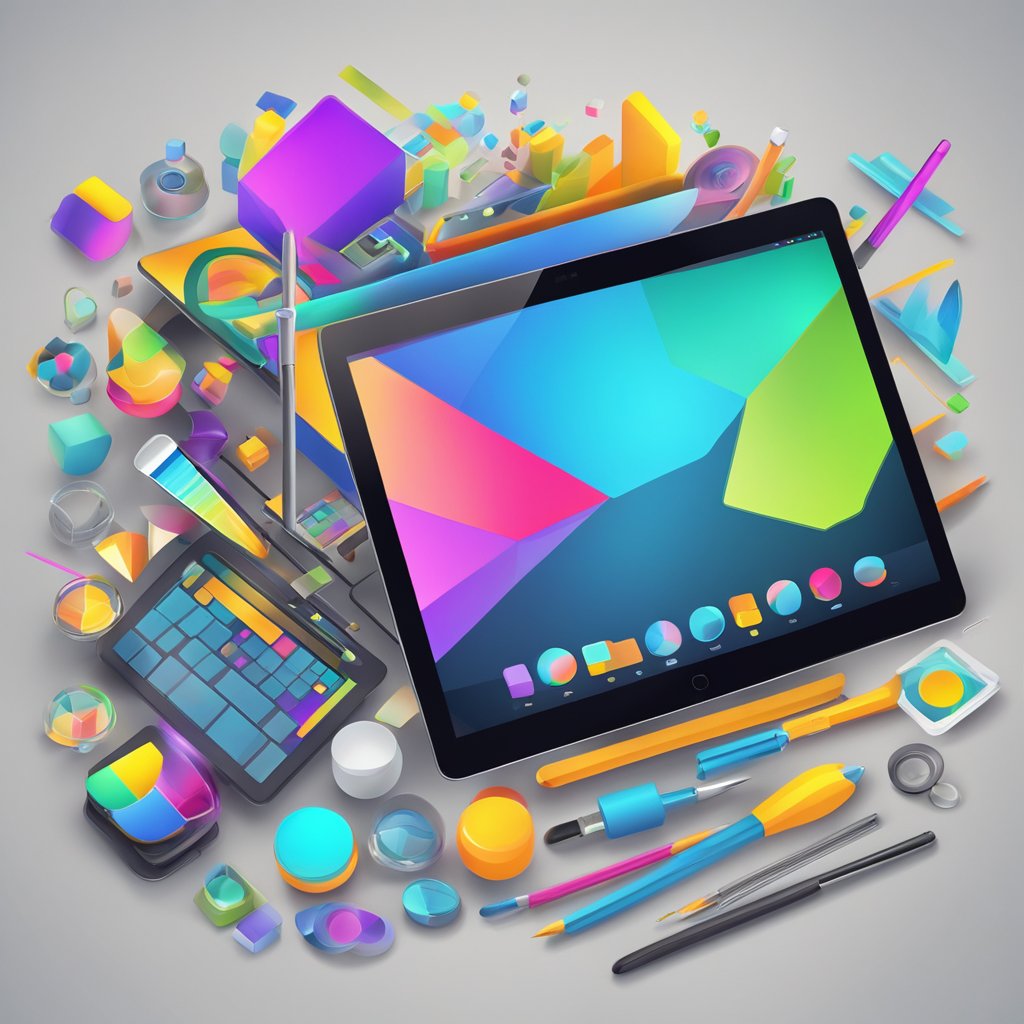
Cómo la tecnología influye en el arte moderno y la creatividad
Explore cómo la innovación en el arte digital está redefiniendo la creatividad, combinando tecnología y expresión para transformar el arte en experiencias.
Continúe LeyendoTambién te puede interesar
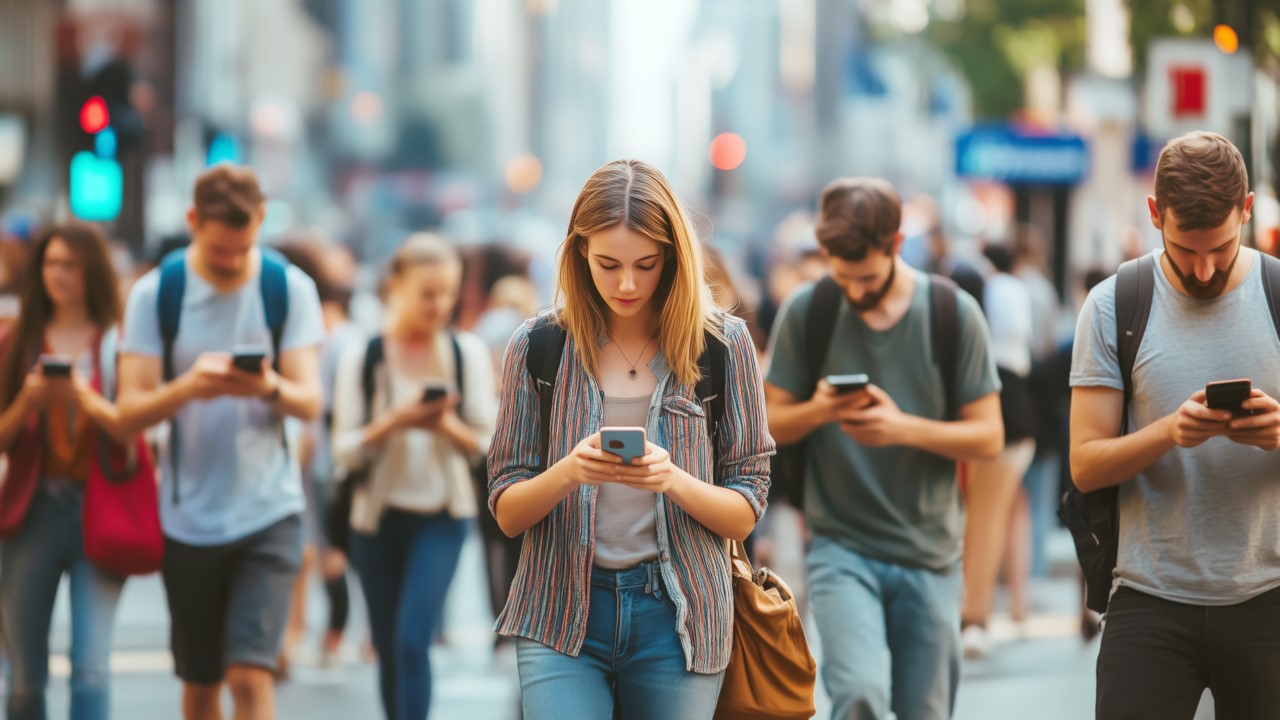
Adicción a la tecnología: estrategias para equilibrar la vida digital con la realidad
Explore estrategias efectivas para combatir la adicción a la tecnología y equilibrar sus interacciones digitales para una mejor salud mental.
Continúe Leyendo